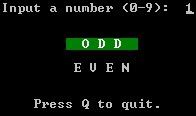
I coded this back when I was taking up Computer System Organization. It was a homework that I decided to share in my old blog.
The program accepts a one-digit number as the input, and then shows whether that number is ODD or EVEN.
The program uses the attribute byte to highlight ODD or EVEN with a color.
.model small
.code
org 100h
start: jmp main
;——– VARS ———
header db “Input a number (0-9): $”
footer db “Press Q to quit.$”
vOdd db “O D D $”
vEven db “E V E N $”
main proc near
mov ax,03 ;clear screen
int 10h
repeat:
call cursor ;header
mov dl,0
mov dh,0
int 10h
mov ah,9
lea dx,header
int 21h
call cursor ;footer
mov dl,4
mov dh,8
int 10h
mov ah,9
lea dx,footer
int 21h
call dispODD
call dispEVEN
call cursor ;input
mov dl,23
mov dh,0
int 10h
call input
cmp al,30h ;0
je pEven
cmp al,31h ;1
je pOdd
cmp al,32h ;2
je pEven
cmp al,33h ;3
je pOdd
cmp al,34h ;4
je pEven
cmp al,35h ;5
je pOdd
cmp al,36h ;6
je pEven
cmp al,37h ;7
je pOdd
cmp al,38h ;8
je pEven
cmp al,39h ;9
je pOdd
cmp al,’Q’
je terminate
cmp al,’q’
je terminate
pEven:
call prEven
jmp repeat
call exit
pOdd:
call prOdd
jmp repeat
call exit
terminate:
call endCursor
call exit
dispODD proc
call cursor ;display ODD
mov dl,10
mov dh,3
int 10h
mov ah,9
lea dx,vOdd
int 21h
ret
dispODD endp
dispEVEN proc
call cursor ;display EVEN
mov dl,9
mov dh,5
int 10h
mov ah,9
lea dx,vEven
int 21h
ret
dispEVEN endp
prEven proc
mov al,’ ‘
mov cx,1
mov bl,2fh
mov dh,5
x: mov dl,8
mov ah,2
int 10h
push cx
mov ah,9
mov cx,9
int 10h
pop cx
inc dh
loop x
call dispEVEN
mov al,’ ‘ ;cover odd
mov cx,1
mov bl,07h
mov dh,3
a: mov dl,8
mov ah,2
int 10h
push cx
mov ah,9
mov cx,9
int 10h
pop cx
inc dh
loop a
call dispODD
ret
prEven endp
prOdd proc
mov al,’ ‘
mov cx,1
mov bl,2fh
mov dh,3
y: mov dl,8
mov ah,2
int 10h
push cx
mov ah,9
mov cx,9
int 10h
pop cx
inc dh
loop y
call dispODD
mov al,’ ‘ ;cover even
mov cx,1
mov bl,07h
mov dh,5
b: mov dl,8
mov ah,2
int 10h
push cx
mov ah,9
mov cx,9
int 10h
pop cx
inc dh
loop b
call dispEVEN
ret
prOdd endp
main endp
;—Program coded by Catzie Keng of http://catzie.net
cursor proc
mov ah,2
mov bh,0
ret
cursor endp
input proc
mov ah,1
int 21h
ret
input endp
endCursor proc
call cursor
mov dl,0
mov dh,15
int 10h
endCursor endp
exit proc
int 20h
exit endp
end start
;—Program coded by Catzie Keng of http://catzie.net
I remember this! 8D
i like it!!
all i can say is not working !!!!!
please check your code before you post it into the internet !
thanks !
All I can say is “Debug it.” It’s not my fault if it won’t work for you. You didn’t see me write “The following code is guaranteed to work on any assembler” did you? Are you looking for code to be used on your homework? Learn by tweaking the codes by yourself.
Please think before you post a rude message!
Thanks!
it worked
Ms. Catzie, can you help me with this errors?
Assembling file: final2.asm
**Error** final2.asm(105) Need address or register
**Error** final2.asm(121) Need address or register
**Error** final2.asm(140) Need address or register
**Error** final2.asm(156) Need address or register
**Fatal** final2.asm(174) Unexpected end of file encountered
Terdapat eror
Assembling file: oddeven.asm
**Error** oddeven.asm(65) Undefined symbol: Q
**Error** oddeven.asm(67) Undefined symbol: Q
**Error** oddeven.asm(107) Need address or register
**Error** oddeven.asm(123) Need address or register
**Error** oddeven.asm(142) Need address or register
**Error** oddeven.asm(158) Need address or register
Error messages: 6
Warning messages: None
Passes: 1
Remaining memory: 440k
mohon pencerahannya
There is an eror in line
Assembling file: oddeven.asm
**Error** oddeven.asm(65) Undefined symbol: Q
**Error** oddeven.asm(67) Undefined symbol: Q
**Error** oddeven.asm(107) Need address or register
**Error** oddeven.asm(123) Need address or register
**Error** oddeven.asm(142) Need address or register
**Error** oddeven.asm(158) Need address or register
Error messages: 6
Warning messages: None
Passes: 1
Remaining memory: 440k
please give me some information
hello…
im a BSIT student and im worried to pass this subject..
help me please to this output:
Output: Enter Number: 4
1
12
123
1234
please help me..
here’s my email add:
[email protected]
is this code for masm6.11 ???
a girl doing assembly? sexy! but here’s the criticism – you actually comapre each digit so see if it’s even or odd!? what if you where to rewrite it for number 1-100? there’s smarter ways.
hi , catzie , i learned how to program , but i don’t know what is assembler and other stuff like Computer Org , pls recommand any books or Resources to learn all stuff .